Blog
Validation - Enforcing minimum and maximum dates with the date picker control
When building data entry screens, it's often necessary to validate dates. Unfortunately, the date picker control contains no built-in way to specify the minimum and maximum permissible values, or other validation rules. This post describes a way to restrict the value that a user can enter through a date picker control.
Date picker - How to set a maximum date (eg Date cannot be greater than today)
To demonstrate how to enforce a maximum date, let's take the example where a user must not enter a 'create date' that is greater than today. To apply this rule, we can apply the following formula to the OnChange property of the date picker control.
If(Self.SelectedDate > Today() ,
Notify("Create date can not be greater than today",
NotificationType.Error);
Reset(Self)
)
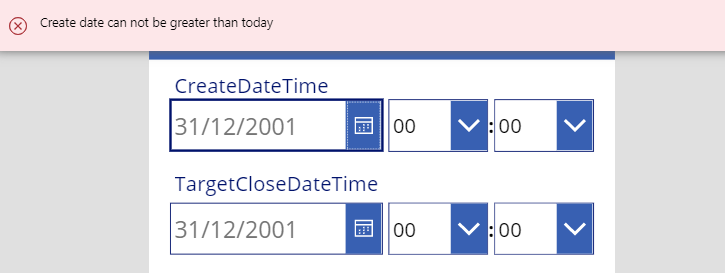
Date picker - How to set a minimum date (eg Date cannot be greater than today)
We can apply the same technique to enforce a minimum date. For example, we can apply
the following formula to the OnChange property of a 'target close date' date picker control. This formula ensures that a user must enter a target close date in the future.
If(Self.SelectedDate < Today() ,
Notify("Target close date can not be less than today",
NotificationType.Error);
Reset(Self)
)
If(Self.SelectedDate < Today() And (EditForm1.Mode = FormMode.New),
Notify("Target close date can not be less than today",
NotificationType.Error);
Reset(Self)
)
Comparing two dates (eg close date cannot be greater than create date)
Another common scenario is to compare an input date against another field value. As an example, we can add the following formula to the OnChange property of a 'close date' date picker control. This formula ensures that a user must enter a 'close date' that is later than the 'create date'.
If(Self.SelectedDate < datePickerCreateDate.SelectedDate,
Notify("Close date can not be earlier than create date",
NotificationType.Error);
Reset(Self)
)